C++:
void buildPyramid(InputArray src, OutputArrayOfArrays dst, int maxlevel, int borderType=BORDER_DEFAULT )
Parameters: |
|
The function constructs a vector of images and builds the Gaussian pyramid by recursively applying
pyrDown()
to the previously built pyramid layers, starting from dst[0]==src
.Reference: OpenCV Documentation - buildPyramid
Example
This is a sample code (C++) with images for opencv build Pyramid filter.
sstring imgFileName = "lena.jpg";
cv::Mat src = cv::imread(imgFileName);
if (!src.data){
cout << "Unable to open file" << endl;
getchar();
return 1;
}
int maxVal = 4;
vector dstVect;
cv::buildPyramid(src, dstVect, maxVal);
cv::namedWindow("Source");
cv::imshow("Source", src);
string imgName;
for (int i = 0; i < maxVal + 1; i++){
stringstream ss;
ss << "Filtered " << i;
imgName = ss.str();
cv::namedWindow(imgName);
cv::imshow(imgName, dstVect[i]);
ss << ".jpg";
imgName = ss.str();
cv::imwrite(imgName, dstVect[i]);
}
cv::waitKey(0);
return 0;
Filtered Image | Source Image |
Image size: 512 x 512 Image size: 256 x 256 Image size: 128 x 128 Image size: 64 x 64 Image size: 32 x 32 |
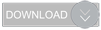
Download complete Visual Studio project.
No comments:
Post a Comment