C++:
void blur(InputArray src, OutputArray dst, Size ksize, Point anchor=Point(-1,-1), int borderType=BORDER_DEFAULT )
Python:
cv2.blur(src, ksize[, dst[, anchor[, borderType]]]) → dst
Parameters: |
|
---|
The function applies bilateral filtering to the input image, as described in http://www.dai.ed.ac.uk/CVonline/LOCAL_COPIES/MANDUCHI1/Bilateral_Filtering.html bilateralFilter can reduce unwanted noise very well while keeping edges fairly sharp. However, it is very slow compared to most filters.
Sigma values: For simplicity, you can set the 2 sigma values to be the same. If they are small (< 10), the filter will not have much effect, whereas if they are large (> 150), they will have a very strong effect, making the image look “cartoonish”.
Filter size: Large filters (d > 5) are very slow, so it is recommended to use d=5 for real-time applications, and perhaps d=9 for offline applications that need heavy noise filtering.
This filter does not work inplace.
Reference: OpenCV Documentation - bilateralFilter
Example
This is a sample code (C++) with images for opencv bilateral filter filter.
string imgFileName = "lena.jpg";
cv::Mat src = cv::imread(imgFileName);
if (!src.data){
cout << "Unable to open file" << endl;
getchar();
return 1;
}
cv::Mat dst;
cv::bilateralFilter(src, dst, 20, 100, 100);
cv::namedWindow("Source");
cv::namedWindow("Filtered");
cv::imshow("Source", src);
cv::imshow("Filtered", dst);
cv::waitKey(0);
cv::imwrite("BilateralFilter.jpg", dst);
return 0;
Filtered Image | Source Image |
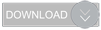
Download complete Visual Studio project.
No comments:
Post a Comment