C++:
void boxFilter(InputArray src, OutputArray dst, int ddepth, Size ksize, Point anchor=Point(-1,-1), bool normalize=true, int borderType=BORDER_DEFAULT )
Python:
cv2.boxFilter(src, ddepth, ksize[, dst[, anchor[, normalize[, borderType]]]]) → dst
Parameters: |
|
---|
The function smoothes an image using the kernel:
Where,
Unnormalized box filter is useful for computing various integral characteristics over each pixel neighborhood, such as covariance matrices of image derivatives (used in dense optical flow algorithms, and so on). If you need to compute pixel sums over variable-size windows, use integral().
Reference: OpenCV Documentation - boxFilter
Example
This is a sample code (C++) with images for opencv box filter.
string imgFileName = "lena.jpg";
cv::Mat src = cv::imread(imgFileName);
if (!src.data){
cout << "Unable to open file" << endl;
getchar();
return 1;
}
cv::Mat dst;
cv::boxFilter(src, dst, -1, cv::Size(16, 16));
cv::namedWindow("Source");
cv::namedWindow("Filtered");
cv::imshow("Source", src);
cv::imshow("Filtered", dst);
cv::waitKey(0);
cv::imwrite("Box Filter.jpg", dst);
return 0;
Filtered Image | Source Image |
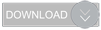
Download complete Visual Studio project.
No comments:
Post a Comment