AForge.NET is a powerful C# framework designed for the fields of Computer Vision and Artificial Intelligence, image processing, neural networks, genetic algorithms, machine learning, robotics, etc.
This is a simple and quick tutorial that describes how to setup Visual Studio environment to work with AForge.net. At the end of this tutorial you will have a C# project with all the required dll files for AForge.net and a simple few line code to convert RBG C# Bitmap image to grayscale image.
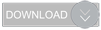
Download complete Visual Studio project.
Step 1 - Create new project
Open Visual Studio IDE and create a new project, Goto File-->New-->ProjectCreate a C# winform Application project by selecting Windows Forms Application from the list and give it a proper name and folder to save the project
Step 2 - Add references for AForge.net
Open solution explorer and right click on your project then select Manage NuGet Packages...This will open a new window to manage your references via NuGet. NuGet is a nice way to keep your references, But this required your computer connected to internet. Therefore make sure your dev machine connected to internet when you try next step.
Now, simply search for aforge on the search box and click on install button on the AForge.Imaging library. So this will take few seconds to download all the dependencies and the library itself to your project.
Note that the coolest thing in the NuGet is that you do not need to worry about dependencies of a library, it will take care of all required libraries for the one that you want to install.
After you are done with the installation, your References for the project will looks like this,
Step 3 - Create winform
Now, let's do some UI to do our magic. Click on tool bar and draw a button. You can give it a proper name and text.I called my button as Process and named it as btnProcess. So let's make some code in the next step.
Step 4 - Code it !!!
Double click on the Process button in your winform designer and Visual Studio will open the code view and automatically add a Click event for your button. So let's do our coding inside the click event, then it will execute the code when you click on the Process button.Since this code needs to convert an RGB image to grayscale, we need to apply a filter on AForge.net called Grayscale. Therefore we need to create the filter first.
Type Grayscale in the code and Visual Studio will give you an error says that "The type or namespace name 'Grayscale' could not be found (are you missing a using directive or an assembly reference?)". That's mean, we didn't import AForge namespace to this file still.
To fix this error, either you can type
using AForge.Imaging.Filters;
or Right on Grayscale and select Resolve-->using AForge.Imaging.Filters;Either case your code should be like this and note that you will have
using AForge.Imaging.Filters;
at the top of your file.Now, type the following code to open an image and apply the grayscale filter and save it back to the disk.
Grayscale filter = new Grayscale(0.2, 0.2, 0.2);
Bitmap img = (Bitmap)Image.FromFile("test.jpg");
Bitmap grayImage = filter.Apply(img);
grayImage.Save("grayImage.jpg");
Code Explained...
Grayscale filter = new Grayscale(0.2, 0.2, 0.2);
Bitmap img = (Bitmap)Image.FromFile("test.jpg");
System.Drawing.Bitmap
type object. Note that, if your image is in a different path, you need to type the full path of the jpg file instead of test.jpg.Bitmap grayImage = filter.Apply(img);
img
is our source image and this will return a filter applied Bitmap type object. So grayImage
is the output image.grayImage.Save("grayImage.jpg");
Now, you can build your project. Go to Build-->Build Solution from the main menu.
If every thing went well without any error in your code, you may see success on the Output window as follows,
Step 5 - One more before run...
Note that all the path for our jpg files are relative paths, which means that is not a the full path to the file. So this means all the paths are relative the directory where our exe file located on, in this case it will located on your bin/debug folder of the Visual Studio project. Therefore go to your project folder and put test.jpg file in the bin/debug folder as shown here,Step 6 - Run the code
Ok, Now your project is ready to run and test. so go to Debug-->Start Debugging or press F5 to run your project.Then you will see your winform and click on process button.
If every thing went well, you will see a jpg file in your bin/debug folder named grayImage.jpg and it is the grayscale image for the test.jpg image.
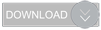
Download complete Visual Studio project.
Filtered Image | Source Image |
c'est vraiment très cool
ReplyDeleteMilinda Pro: Simple Aforge.Net Tutorial - Getting Start With Aforge >>>>> Download Now
Delete>>>>> Download Full
Milinda Pro: Simple Aforge.Net Tutorial - Getting Start With Aforge >>>>> Download LINK
>>>>> Download Now
Milinda Pro: Simple Aforge.Net Tutorial - Getting Start With Aforge >>>>> Download Full
>>>>> Download LINK QQ
Milinda Pro: Simple Aforge.Net Tutorial - Getting Start With Aforge >>>>> Download Now
ReplyDelete>>>>> Download Full
Milinda Pro: Simple Aforge.Net Tutorial - Getting Start With Aforge >>>>> Download LINK
>>>>> Download Now
Milinda Pro: Simple Aforge.Net Tutorial - Getting Start With Aforge >>>>> Download Full
>>>>> Download LINK 4b
Good content. You write beautiful things.
ReplyDeletesportsbet
vbet
vbet
mrbahis
hacklink
sportsbet
korsan taksi
taksi
hacklink
Success Write content success. Thanks.
ReplyDeletebetmatik
betpark
deneme bonusu
kıbrıs bahis siteleri
canlı poker siteleri
canlı slot siteleri
kralbet
Good content. You write beautiful things.
ReplyDeletemrbahis
sportsbet
hacklink
vbet
sportsbet
taksi
korsan taksi
mrbahis
vbet
dijital kartvizit
ReplyDeletereferans kimliği nedir
binance referans kodu
referans kimliği nedir
bitcoin nasıl alınır
resimli magnet
YGP
التخلص من رائحة المجاري في المطبخ A5XOwPUEor
ReplyDelete